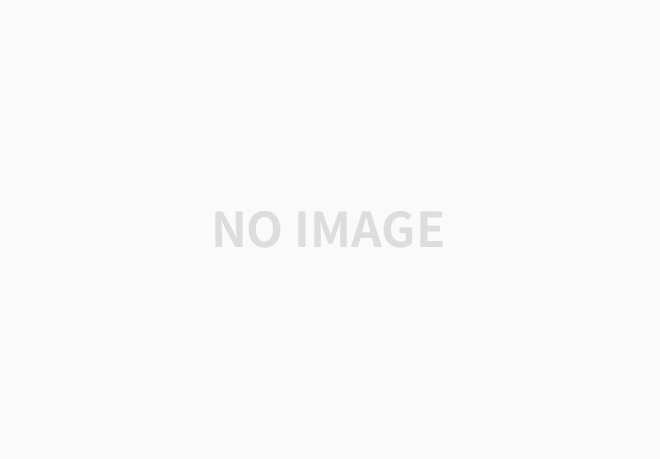
Typography 설정
1. 폰트 파일 추가
res/font 폴더에 파일 추가
소문자와 언더바(_)만 사용 가능
app/
└ res/
└ font/
└ hakgyoansimalrimche.ttf
2. Font.kt 생성
경로 : app/src/main/java/com/example/picktimeapp/ui/theme/Font.kt
package com.example.picktimeapp.ui.theme
import androidx.compose.ui.text.font.Font
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.text.font.FontWeight
import com.example.picktimeapp.R
// Pretendard FontFamily
val Pretendard = FontFamily(
Font(R.font.pretendard_thin, FontWeight.Thin),
Font(R.font.pretendard_extralight, FontWeight.ExtraLight),
Font(R.font.pretendard_light, FontWeight.Light),
Font(R.font.pretendard_regular, FontWeight.Normal),
Font(R.font.pretendard_medium, FontWeight.Medium),
Font(R.font.pretendard_semibold, FontWeight.SemiBold),
Font(R.font.pretendard_bold, FontWeight.Bold),
Font(R.font.pretendard_extrabold, FontWeight.ExtraBold),
Font(R.font.pretendard_black, FontWeight.Black)
)
// 학교안심알림체 FontFamily
val TitleFont = FontFamily(
Font(R.font.hakgyoansimalrimche_regular, FontWeight.Normal),
Font(R.font.hakgyoansimalrimche_bold, FontWeight.Bold)
)
3. 전역 Typography 설정(Type.kt)
package com.example.picktimeapp.ui.theme
import androidx.compose.material3.Typography
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.unit.sp
val AppTypography = Typography(
displayLarge = TextStyle(
fontFamily = TitleFont,
fontWeight = FontWeight.Bold,
fontSize = 92.sp
),
titleLarge = TextStyle(
fontFamily = Pretendard,
fontWeight = FontWeight.Bold,
fontSize = 84.sp
),
titleMedium = TextStyle(
fontFamily = Pretendard,
fontWeight = FontWeight.SemiBold,
fontSize = 72.sp
),
bodyLarge = TextStyle(
fontFamily = Pretendard,
fontWeight = FontWeight.Normal,
fontSize = 52.sp
),
bodyMedium = TextStyle(
fontFamily = Pretendard,
fontWeight = FontWeight.Normal,
fontSize = 40.sp
),
bodySmall = TextStyle(
fontFamily = Pretendard,
fontWeight = FontWeight.Normal,
fontSize = 28.sp
)
)
참고 ) 1px은 몇 sp?
1px와 sp의 관계는 디스플레이의 해상도(dpi)에 따라 달라진다
px
screen pixels을 나타내는 단위로 실제 크기나 밀도에 관계없이 고정된 단위이다
다양한 디바이스가 있으므로 절대적인 사이즈인 px 사용은 지양해야 한다
sp
Scalable Pixels로, Scale 값을 조절할 수 있도록 픽셀을 표현하는 단위시스템에서 설정한 폰트 사이즈에 영향을 받는 단위
dp
안드로이드 디바이스는 다양한 해상도가 존재하기 때문에, 다양한 환경을 지원하기 위해 만들어진 단위이다
레이아웃 등 뷰가 모든 디바이스에서 동일한 비율을 가지기 위해서는 dp를 사용해야 한다
dp가 안드로이드 뷰에서 사용하는 단위라면 sp는 안드로이드 글자에서 사용하는 단위 개념
4. Theme.kt
@Composable
fun PickTimeAppTheme(
dynamicColor: Boolean = true,
content: @Composable () -> Unit
) {
val colorScheme = LightColorScheme // 생략
MaterialTheme(
colorScheme = colorScheme,
typography = AppTypography, // 👈 폰트 적용
content = content
)
}
사용해보기
전역 폰트 사용
Text(text = "전역 Pretendard 적용됨") // 아무 설정 없어도 Pretendard 적용됨
특정 컴포넌트에 폰트 적용
Text(
text = "피크 타임",
fontFamily = HakgyoFont,
fontWeight = FontWeight.Bold,
fontSize = 64.sp
)
사이즈가 적용된 스타일로 적용
Text(
text = "피크 타임",
style = MaterialTheme.typography.displayLarge
)
스타일 추가하기
Text(
text = "즐겁고 재미있는 기타 학습",
style = MaterialTheme.typography.bodyMedium,
modifier = Modifier.fillMaxWidth(),
textAlign = TextAlign.End
)
'개발새발개발 > Kotlin' 카테고리의 다른 글
[Kotlin] 안드로이드 스튜디오 프론트엔드가 백엔드와 API 통신하는 법(로그인 구현) (1) | 2025.03.27 |
---|---|
[Kotlin] 안드로이드 스튜디오 배경색, 기본 컬러 지정하는 법 (0) | 2025.03.25 |
[Kotlin] 안드로이드 스튜디오 기본 컬러 정의하는 법(기본, Jetpack) (0) | 2025.03.23 |
[Kotlin] 안드로이드 스튜디오 프로젝트 기본 구조 이해하기 (0) | 2025.03.17 |
[Kotlin] 안드로이드 앱의 구조 및 동작 원리, 자바가 아닌 코틀린을 사용하는이유 (1) | 2025.03.16 |